Slicing is extraction of a part of a sequence like list, tuple, string. It can fetch multiple elements with a single statement. It does not remove the values from the iterable; instead makes a new object with the desired elements.
Syntax
<iterable>[<start>:<end>:<step>]
start – Starting integer where the slicing of the object starts. Default to None
if not provided.
end– Integer until which the slicing takes place. The slicing ends at index end – 1 (last element).
step– Integer value which determines the increment between each index for slicing. Defaults to None
if not provided.
- for zero or positive value of start/end, elements are counted from the start of the iterable.
- for negative value of start/end, elements are counted from the end of the iterable (for e.g.-1 is the last element, -2nd to last etc).
- If step value is 0, then it is set as 1.
- If step value is negative, then the elements are selected in reverse order.
When start and end values are not provided colon must be used instead.
Default value of start and end
- When start value is not provided – Default value of start depends on step size. When step value is positive or omitted, it defaults to the start of the iterable and if step value is negative, start defaults to the end of the iterable.
- When end value is not provided – Similar to start, default value of end depends on step size. When step value is positive or omitted, it defaults to the end of the iterable and if step value is negative it defaults to the start of the iterable.
Let’s create a list to show slicing and Slice at position 3 to 6.
#Creating a list
num_lst = [10,20,30,40,50,60,70,80,90,100]
#Start the slice object at position 3, and slice to position 5, and return the result:
x = slice(3:6)
#Output>>>
[40, 50, 60]
Basic usage of slicing
Python supports slice notation for any sequential data like lists, strings, tuples and ranges. Slicing is a flexible tool to build new sequential from the existing one. It takes start, end and step as an argument. Each of them has a default value as described above. Because of its ease and simplicity it is also popular in data science libraries like NumPy and Pandas.
#Creating a list
num_lst = [10,20,30,40,50,60,70,80,90,100]
#If start is not provided but end and step value are provided
print(num_lst[:5:2])
#Output
>>[10, 30, 50]
#If start and end values are not provided but steps value is provided
print(num_lst[::2])
#Output
>>[10, 30, 50, 70, 90]
#If start and steps are not provided but end value is provided
print(num_lst[:5])
#Output>>>
[10, 20, 30, 40, 50]
#If end is not provided but start and steps values are provided
print(num_lst[3::2])
#Output>>>
[40, 60, 80, 100]
#If end and steps are not provided but start value is provided
print(num_lst[3::])
#Output>>>
[40, 50, 60, 70, 80, 90, 100]
When given values are out of range
#If a position that exceeds the number of elements
print(num_lst[2:20]) #It will not give error, but take the end of the iterable
#Output>>
[30, 40, 50, 60, 70, 80, 90, 100]
If output returns, no elements
When provided values of start and end returns no element, then it will return as an empty list.
print(num_lst[10:20]) #It will not give error
#Output>>>
[]
print(num_lst[2:2]) #It will not give error
#Output>>>
[]
print(num_lst[5:2])
#Output>>>
[]
Using negative indexing
Use negative index to refer element position with respect to end of the list. For e.g. -1 index refers to the last element of the list.
#If end value is negative
print(num_lst[4:-1])
#Output>>>
[50, 60, 70, 80, 90]
Here -1 refers to the last element and as the end element is not include, so it selects the elements till the second last element.
#If start is negative
print(num_lst[-3:]) # This selects the last three elements
#Output>>>
[80, 90, 100]
#If start, end and steps are negative
print(num_lst[-2:-7:-3])
#Output>>>
[90, 60]
#If start and steps are negative
print(num_lst[-2::-3])
#Output>>>
[90, 60, 30]
Getting a list in reverse order
If step value is negative then the elements will selected in reverse order.
#If start and end value are not provided then it will return all element in reversed order
print(num_lst[::-1])
#Output>>>
[100, 90, 80, 70, 60, 50, 40, 30, 20, 10]
#If Step value is negative
print(num_lst[5:2:-1])
#Output>>>
[60, 50, 40]
#If start value is after the steps, it will return an empty list
print(num_lst[2:5:-1])
#Output
[]
Get substring using slice object
The slice object is used to slice a sequence (i.e. string, list, tuple, set or range). This is used for extended slicing. If only one value is passed then start and step value is considered as none i.e. start will default to starting of the iterable and step will default to 1.
# Getting a substring from the given string
string_var = 'PickupBrain'
# If stop = 6
slice_object = slice(6)
print(string_var[slice_object])
#Output>>>
Pickup
# start = 1, stop = 6, step = 2
slice_object = slice(2, 7, 2)
print(string_var[slice_object])
#Output>>>
cuB
Substitute part of a list
Slicing notation can also be used to assign elements.
#Creating a list
num_lst = [10,20,30,40,50,60,70,80,90,100]
num_lst[:5] = [1,2,3,4,5]
print(num_lst)
#Output>>>
[1, 2, 3, 4, 5, 60, 70, 80, 90, 100]
Replace and Resize part of the list
In this case we extend the original list.
num_lst = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
# Replacing first four element of list with bigger list
num_lst[:4] = [1,2,3,4,5,6,7]
print(num_lst)
#Output>>>
[1, 2, 3, 4, 5, 6, 7, 50, 60, 70, 80, 90, 100]
Itβs also possible to replace bigger sub-list with a smaller sub-list:
num_lst = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
num_lst[:4] = [7]
print(num_lst)
#Output>>>
[7, 50, 60, 70, 80, 90, 100]
Replace Every n-th Element
Following code will replace every 2nd element with a ‘*’:
num_lst = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
num_lst[::2] = ['*', '*', '*', '*', '*']
print(num_lst)
#Output>>>
['*', 20, '*', 40, '*', 60, '*', 80, '*', 100]
Using slice assignment with step sets a limitation on the list we provide for assignment. The provided list should exactly match the number of elements to replace. If the length does not match, Python throws the error ValueError: attempt to assign sequence of size 4 to extended slice of size
num_lst = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
num_lst[::2] = [1, 2,3,4]
print(num_lst)
#Output>>>
ValueError: attempt to assign sequence of size 4 to extended slice of size 5
We can use negative step too
num_lst = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
num_lst[::-2] = [1,2,3,4,5]
print(num_lst)
#Output>>>
[10, 5, 30, 4, 50, 3, 70, 2, 90, 1]
By providing start and stop values
num_lst = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
num_lst[1:5:2] = [4, 6]
print(num_lst)
#Output>>>
[10, 4, 30, 6, 50, 60, 70, 80, 90, 100]
Please write comments if you find anything incorrect, or you want to share more information about the topic discussed above
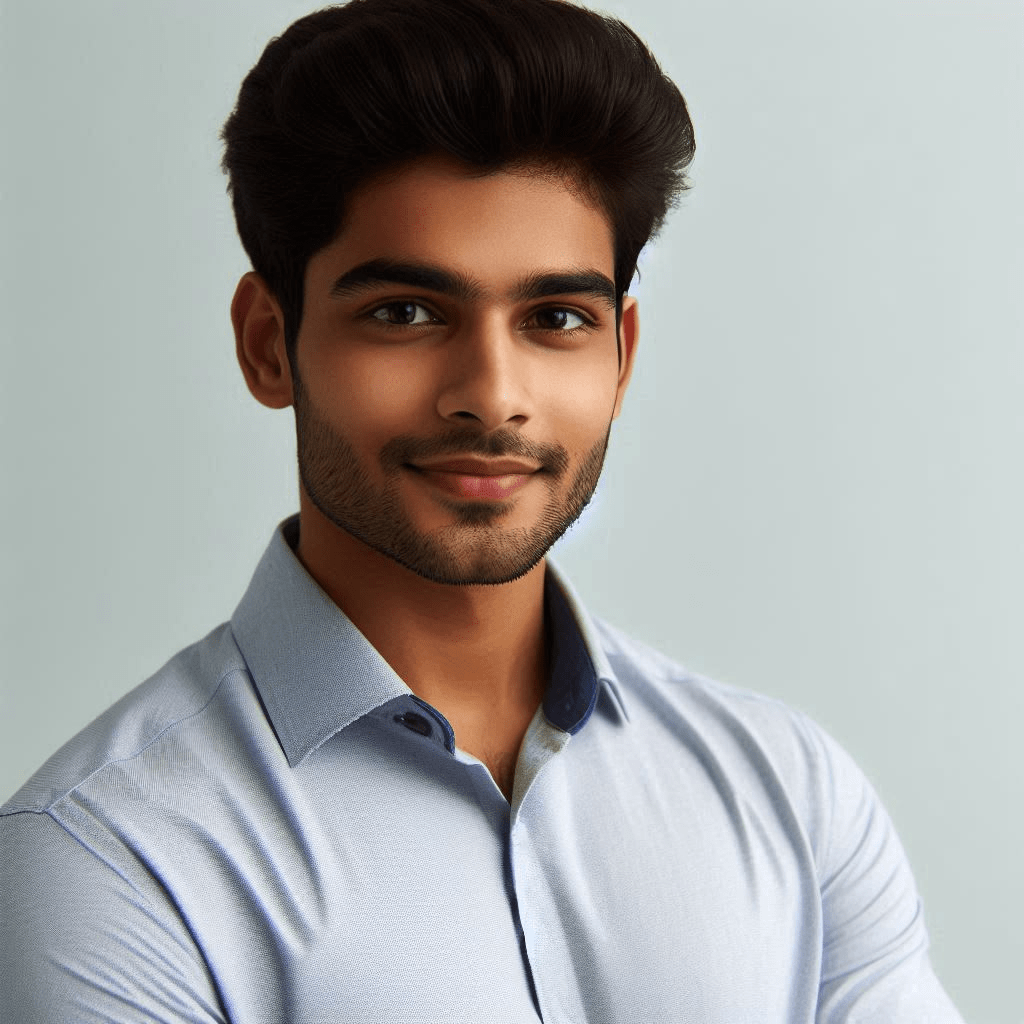
C P Gupta is a YouTuber and Blogger. He is expert in Microsoft Word, Excel and PowerPoint. His YouTube channel @pickupbrain is very popular and has crossed 9.9 Million Views.