Before making a list in Python, let’s understand about it.
What is List?
List is Python’s most flexible ordered collection object type. Unlike strings, lists can contain any sort of object: numbers, string, and even other lists.
Lists are a useful tool for preserving a sequence of data and further iterating over it.
The important characteristics of Python lists are:
- List is ordered collection of object and can be accessed by index.
- List can contain any arbitrary objects.
- List can be nested to arbitrary depth. A list can contain sub-list, which also contain sub-list themselves and so on to the arbitrary depth.
- Lists are mutable. Its content can be changed unlike tuple, which are non-mutable.
- Lists are dynamic.
How to Make a List in Python
List in Python can be created by placing the sequence of data inside the square brackets []. It can have any number of items and they may be of different types(integer, float, string etc.). We can also make an empty list in python.
Following code will show the syntax of list in python.
Creating an Integer List
list_integer = [1,2,3]
print("List of Integer:",list_integer)
The output will be this.
Output:
List of Integer: [1, 2, 3]
Creating an Float List
list_float = [2.3,5.6,9.8]
print("List of Float:",list_float)
The output will be this.
Output:
List of Float: [2.3, 5.6, 9.8]
Creating a String List
list_string = ["Learn Python", 'Pickupbrain']
print("List of String:",list_string)
The Output will be this.
Output:
List of String: ['Learn Python', 'Pickupbrain']
Creating a Mixed List
Unlike other programming language (like C/C++, Java and C#), a Python list can contain data with different data types.
list_mixed = [1,2,3,'Hello',6.7]
print("List of Mixed values:",list_mixed)
The output will be this.
Output:
List of Mixed values: [1, 2, 3, 'Hello', 6.7]
Creating an Empty List
Some time is better to create an empty list and then populate it later using methods like append.
list_empty = []
print("Empty List:",list_empty)
The output will be this.
Empty List: []
Adding an element in a list
To add an element in a list, there are three methods:
- Append() Method:
It adds an element to the end of the list. It does not return the new list but instead modifies the original.
list.append(element) - Extend() Method:
It iterates over its argument by adding each element to the list and extending the list.
list1.extend(list2) - Insert() Method:
It inserts an element to the list at a given index. The index() method searches for an element in the list and returns its index.
list.insert(index, element)
Conclusions
To summarize, in this post we discussed list object in python. We also gave an example of listing in an Integer, Float, String and mixed data types.
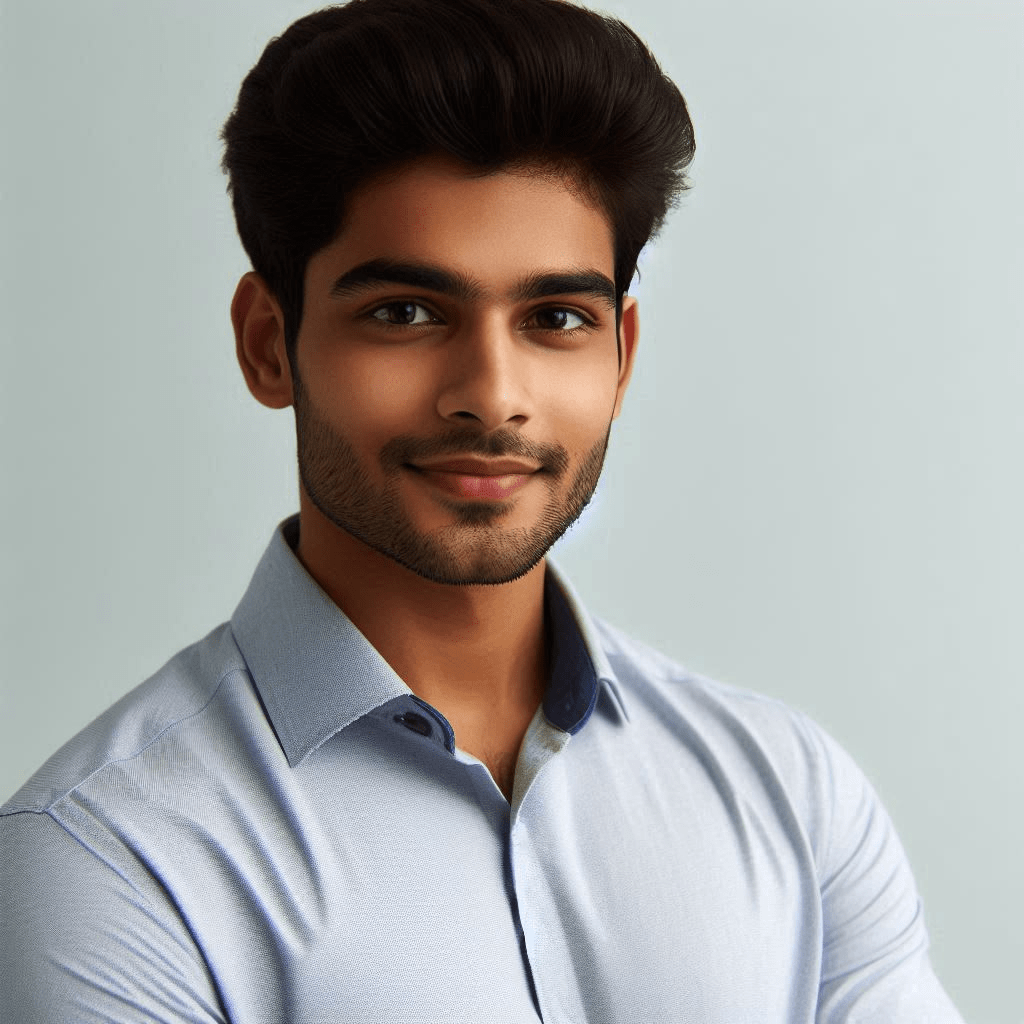
C P Gupta is a YouTuber and Blogger. He is expert in Microsoft Word, Excel and PowerPoint. His YouTube channel @pickupbrain is very popular and has crossed 9.9 Million Views.