The insert() method inserts an element to the list at a given index. The index() method searches for an element in the list and returns its index.
Syntax of Insert() Method
list.insert(index, element)
Insert() Parameters
This method takes two parameter
- index where an element needs to be inserted
- element is the element to be inserted in the list.
This method doesn’t return any value. It just insert an element at the given index.
Inserting Element to List
# Creating a list
list_example = ['Pickupbrain', 'Well', 'Python']
# Inserting element to list at 2nd position
list_example.insert(1, 'Learn')
print('Updated List: ', list_example)
The output will be:
Output:
Updated List: ['Pickupbrain', 'Learn', 'Well', 'Python']
Inserting a Tuple to the List
#Creating a list
tuple_data = [{1, 2}, [5, 6, 7]]
# Creating a list with integer
integer_data = (3, 4)
# Inserting tuple to the list
tuple_data.insert(1, integer_data)
print('Updated List: ', tuple_data)
The output will be
Updated List: [{1, 2}, (3, 4), [5, 6, 7]]
As you can see, given index is 1, but it inserts an element in 2nd position because the index in Python starts from 0, not 1. I
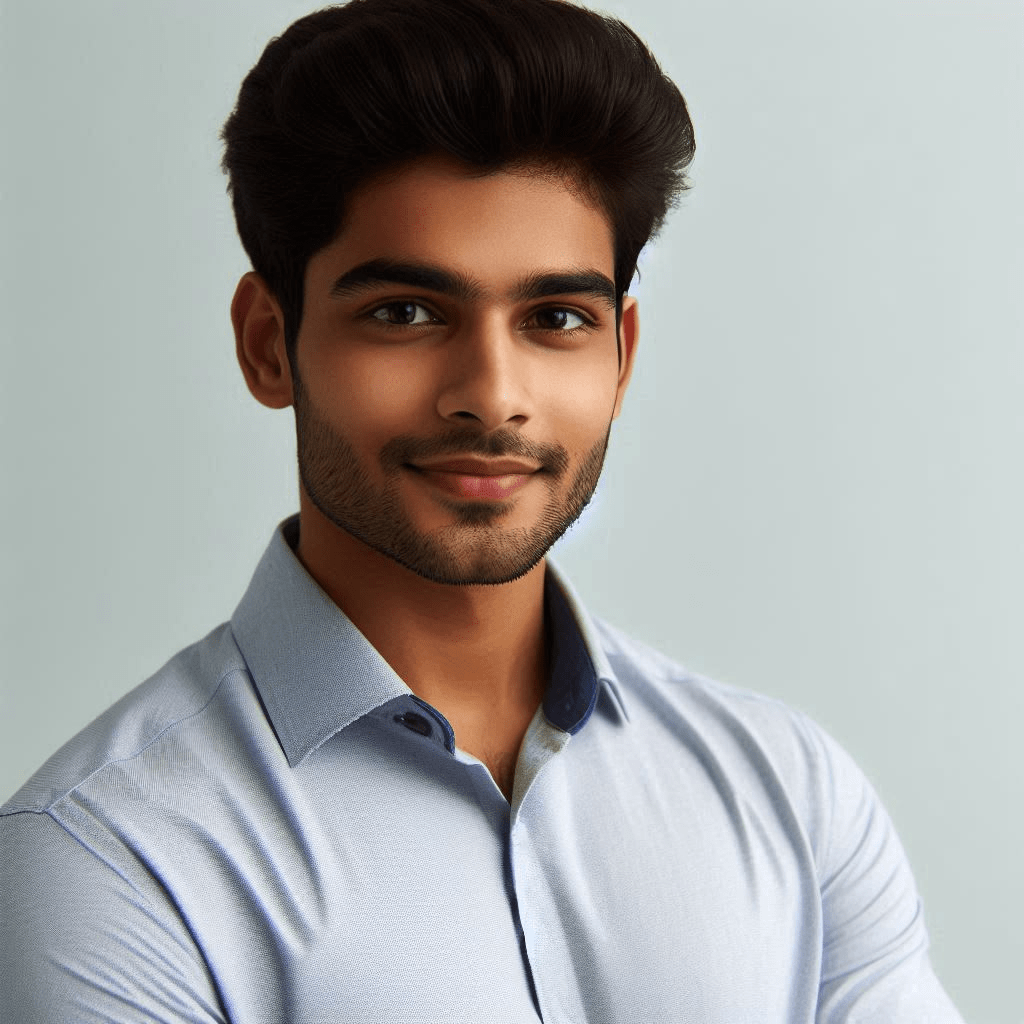
C P Gupta is a YouTuber and Blogger. He is expert in Microsoft Word, Excel and PowerPoint. His YouTube channel @pickupbrain is very popular and has crossed 9.9 Million Views.