Understanding List is one of the most important thing for anyone who just started coding in Python. You may need to add an element to the list whether at the end or somewhere in between. Although it can be done manually with few lists of code, built in function come as a great time saver. There are 3 in-build method to add an element in a list. Beginners sometimes got confused with Append, Extend and Insert methods while working with the list in Python.
Following image summarizes all the three methods and what they do.
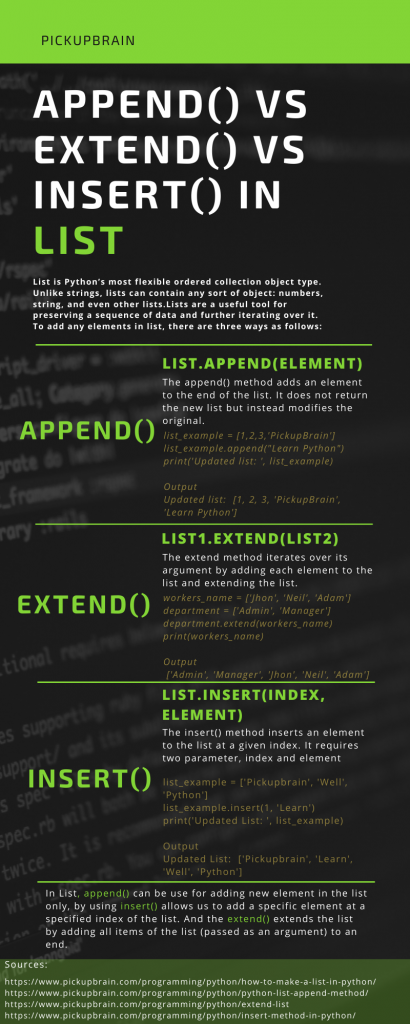
Append() Method
The append method adds a single element or a group of element to the existing list. It always adds an element at the end of the list. When you try to append a list to another list using append method, the entire list is appended as a single element at the end of the original list. For more details about this method please visit append() method
Syntax
list.append(element)
Example
#Creating a list
case_1 = [10,20,30,40,50]
print("The List is: ", case_1)
#Adding an element, using append
case_2 = 60
case_1.append(case_2)
print("The updated list is: ",case_1)
The output will be
Output:
The List is: [10, 20, 30, 40, 50]
The updated list is: [10, 20, 30, 40, 50, 60]
Extend() Method
The extend method extends the list by adding all the elements of the passing iterable to the end. This iterable can be list, tuple or string This method is specially useful when you want to all the elements of a list to another list as an individual element. For details on extend() method please visit here
Syntax
list1.extend(list2)
Example
#Creating a list of fruits
fruits_data = ['Apple', 'Banana','Mango','Guava']
print("The fruits list is: ",fruits_data)
#Create a list of veggies
veggie_data = 'Potato', 'Onion', 'Tomato'
print("The veggie list is: ",veggie_data)
#Using Extend method
fruits_data.extend(veggie_data)
print("The updated list is: ",fruits_data)
The output will be
Output:
The fruits list is: ['Apple', 'Banana', 'Mango', 'Guava']
The veggie list is: ('Potato', 'Onion', 'Tomato')
The updated list is: ['Apple', 'Banana', 'Mango', 'Guava', 'Potato', 'Onion', 'Tomato']
Insert() Method
Append and extend method discussed above adds element only at the end of the list. To insert a new element to any position of the list you can use insert method. It inserts the specified value at the specified index. This method doesn’t return any value but takes two parameters:
index: It specifies where an element needs to be inserted.
element: The element to be inserted in the list.
For more details about Insert method please visit here
Syntax
list.insert(index, element)
Example
#Creating a list of fruits
fruits_data = ['Apple', 'Banana','Mango','Guava']
print("The fruits list is: ",fruits_data)
# Inserting an element to list at 2nd position
fruits_data.insert(1, "Orange")
print("The updated list is: ",fruits_data)
The output will be
Output:
The fruits list is: ['Apple', 'Banana', 'Mango', 'Guava']
The updated list is: ['Apple', 'Orange', 'Banana', 'Mango', 'Guava']
Conclusion
Append adds argument as a single element to the end of a list whereas extend iterate over its argument adding each element at the end of list. Both these methods help you insert new elements at the end of the list. However, using insert method you can add a new element at any position of the exiting list.
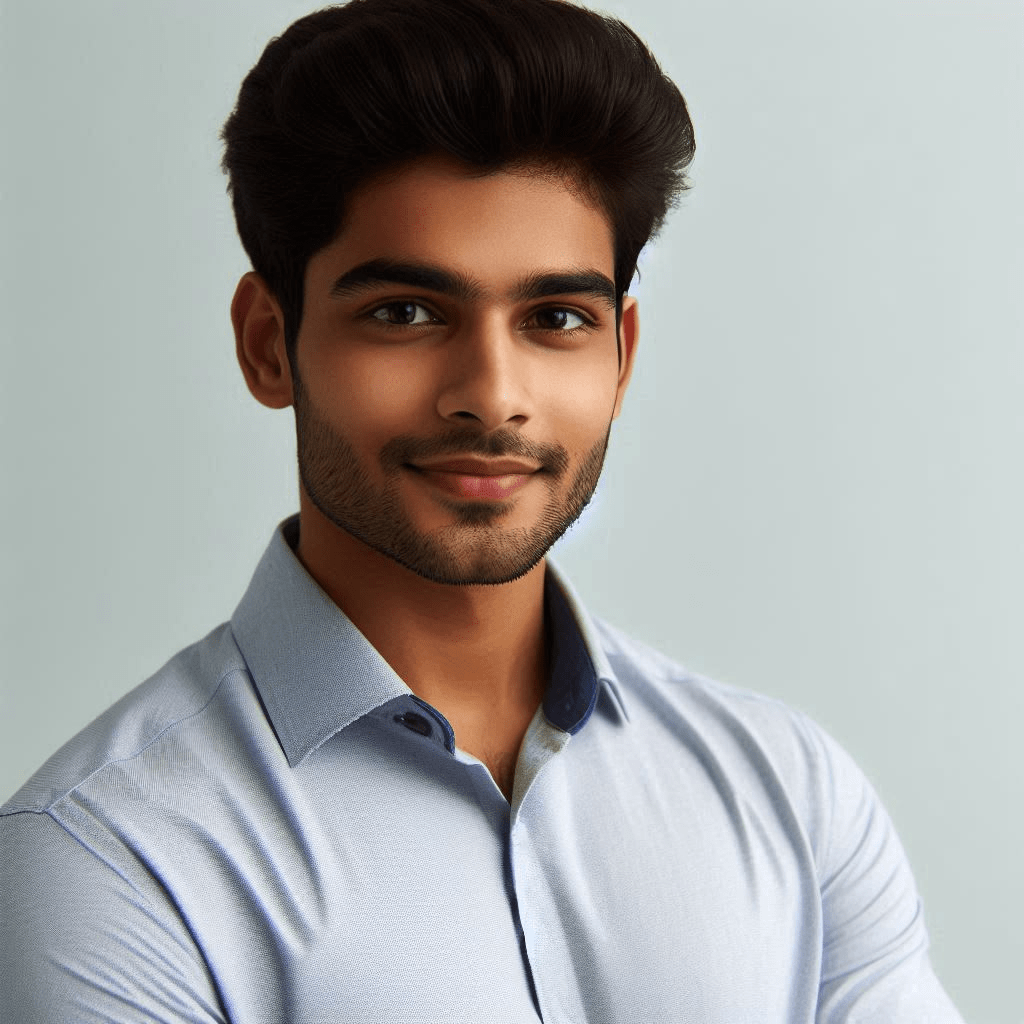
C P Gupta is a YouTuber and Blogger. He is expert in Microsoft Word, Excel and PowerPoint. His YouTube channel @pickupbrain is very popular and has crossed 9.9 Million Views.