In simple words, iterator is an object that can be iterated upon. They contain more than one data in it. Commonly used Python iterators are list, tuple and dictionary. An iterator will return data from that object, one item at a time.
Any Python iterator must implement two methods:
- The __iter__ method which returns the iterator object
- The __next__ method which return the next value for the iterable.
How Does Iteration Works in Python
You can iterate an object by calling the __iter__() method over it. __next__ function is used to the next element of the iterator. When there is no more data to be returned, it raise the StopIteration exception.
In-build Iterators
Examples of inbuilt iterators in Python are list, tuples, dictionaries and string.
#Creating an iterable from Tuple
#Define a list
mytuple = ("PickupBrain", "Learn", "Python")
# Get an iterator using iter()
myiter = iter(mytuple)
# iterate through it using next()
print(next(myiter))
print(next(myiter))
print(next(myiter))
#Output>>
>>>PickupBrain
>>>Learn
>>>Python
#Creating an iterable from List
mylist = [10,20,30,40]
myiter = iter(mylist)
print(next(myiter))
print(next(myiter))
print(next(myiter))
print(next(myiter))
#Output>>
>>>10
>>>20
>>>30
>>>40
#Creating an iterable from String
mystr = "Python"
myit = iter(mystr)
print(next(myit))
print(next(myit))
print(next(myit))
print(next(myit))
print(next(myit))
print(next(myit))
#Output>>
>>>P
>>>y
>>>t
>>>h
>>>o
>>>n
#Creating an iterable from Empty List
my_list = []
empty_element = iter(my_list)
print(next(empty_element))
print(next(empty_element))
#Output>>
>>>Traceback (most recent call last):
>>>File "<ipython-input-20-9ecc7d9f31d7>", line 3, in <module>
>>> print(next(empty_element))
>>>StopIteration
Looping through an Iterator
Using for loop to iterate through an iterable object.
#For loop to iterate my_list
my_list = ("PickupBrain", "Learn", "Python")
for word in my_list:
print(word)
#Output>>>
>>>PickupBrain
>>>Learn
>>>Python
Creating an Iterator
Creating an iterator from scratch is very simple in Python. We just have to use the __iter__ and __next__ methods.
#Creating an iterator that returns numbers, starting with 1, and each sequence will increase by 2 :
class MyNums:
def __init__(self, mx = 20): # default value is 20
self.max = mx
def __iter__(self):
self.a = 1
return self
def __next__(self):
if self.a <= self.max:
x = self.a
self.a += 2
return x
else:
raise StopIteration
my_class = MyNums()
my_iter = iter(my_class)
for x in my_iter:
print(x)
my_class = MyNums()
my_iter = iter(my_class)
for x in my_iter:
print(x)
#Output>>>
1
3
5
7
9
11
13
15
17
19
Difference Between Iterators & Iterables in Python
An object is called iterable if we can get an iterator from it. Lists, tuples, dictionaries and sets are all iterable objects, from which you can get an iterator.
Benefits of Iterators in Python
- Reduce Code Complexity
- Code Redundancy
- Save Resources
Conclusion
Iterator is an object that produces its next value when you call the build-in Python function __next__ on it. They are extremely useful when you need to iterate through a large sequence of items one at a time without having to load all the items into memory at once.
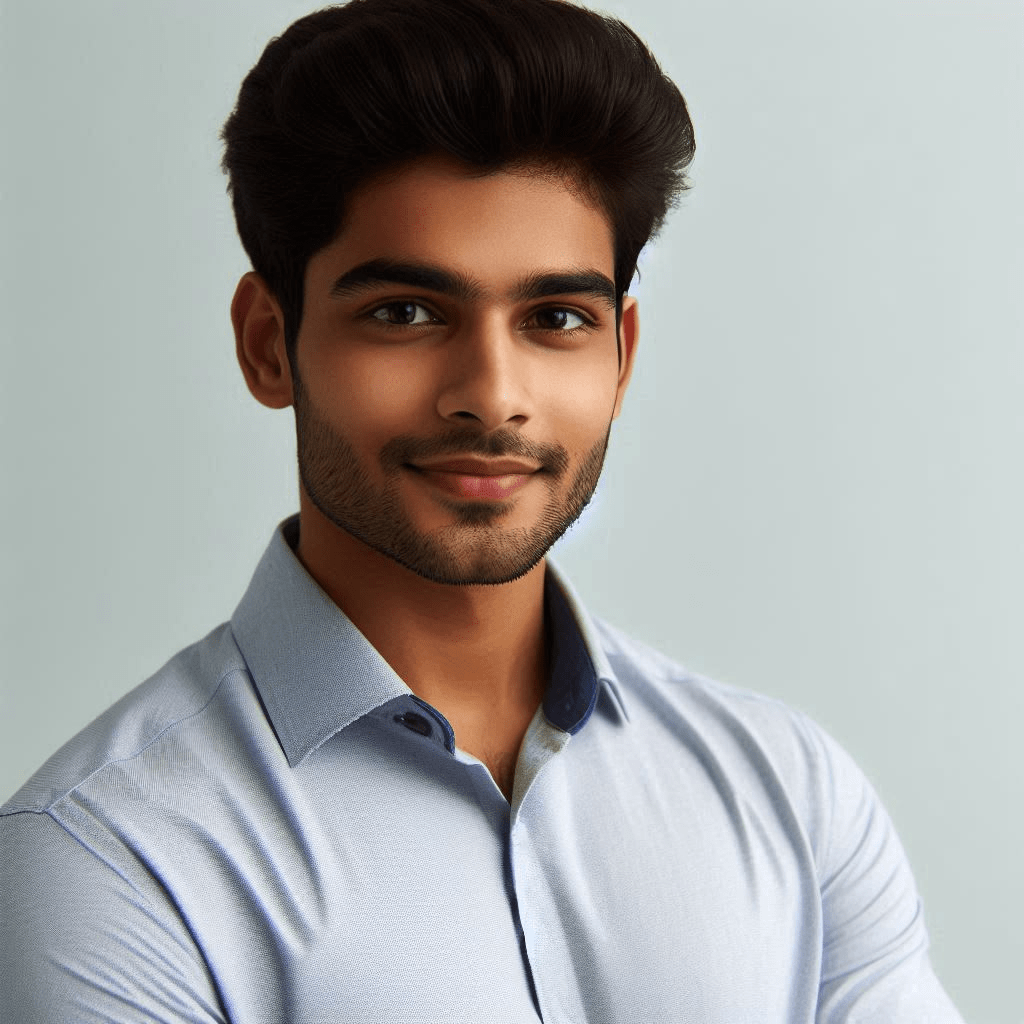
C P Gupta is a YouTuber and Blogger. He is expert in Microsoft Word, Excel and PowerPoint. His YouTube channel @pickupbrain is very popular and has crossed 9.9 Million Views.