A Conditional Statements is a statement that checks for a Boolean condition. It decides whether the block of statement will execute or not. If the condition evaluates to TRUE a section of code will execute.
There are various types of conditional statements in Python, let’s discuss one by one along with their syntax.
Basic Syntax of Conditional Statements
if [conditional]:
[statement(s) to execute]
[conditional] : Any condition based on a combination of one or more variables.
[statement]: is a valid Python statement, which must be indented.
Every conditional statement ends with colon (:), else python will throw an error. Some programming languages require [statement] to be enclosed in parentheses, but Python does not.
The output of the conditional is a Boolean expression, that evaluates to either TRUE or FALSE.
When the conditions is evaluated as TRUE the immediate follow statement block will execute. If conditions evaluates to FALSE then it is skipped and not executed.
if Condition
In almost every program, there are times when you need to make decisions and that’s when you use an if statement. It decides whether certain statements need to be executed or not. If statement checks for a given condition, if the condition is TRUE, then the set of code present inside the if block will be executed.
#if Condition
#if condition is true then
Num1 = 7
Num2 = 10
if(Num1 < Num2):
print("%d is smaller than %d" %(Num1, Num2))
#Output>>>
>>> 7 is smaller than 10
#if condition is false then
Num1 = 7
Num2 = 10
if(Num1 > Num2):
print("%d is greater than %d" %(Num1, Num2))
"""This program won’t provide any output at all. The reason being, the conditional evaluated to be false and hence the print statement was skipped as it was dependent on the conditional."""
There are two more keywords that are optional, but can be accompanied with if
statements, the are:
- else
- elif
else Condition
The statement itself tells that if a given condition is TRUE then execute the statements present inside if block and if the condition is FALSE then execute the else block. Else block will execute only when all the conditions in if/elif statements are evaluated as FALSE.
if [conditional]:
[Statement 1 to be executed if the conditional evaluates to be True]
else:
[Statement 2 to be executed if the conditional evaluates to be False]
In if-else statement, we can diverge the flow of execution into two different directions. The condition will be evaluated to a Boolean expression (TRUE or FALSE). If the condition is TRUE then the statements following if block will be executed and when the condition evaluates to FALSE then the statements following else block will be executed.
#if-else Condition
#if condition is true
Num1 = 7
Num2 = 10
if (Num1 < Num2):
print("%d is smaller than %d" %(Num1, Num2))
else:
print("%d is greater than %d" %(Num1, Num2))
#Output>>>
>>>7 is smaller than 10
#if condition is false
Num1 = 70
Num2 = 10
if (Num1 < Num2):
print("%d is smaller than %d" %(Num1, Num2))
else:
print("%d is greater than %d" %(Num1, Num2))
#Output>>>
>>>70 is greater than 10
elif Condition
The elif condition is added after if condition. Use of else statement with elif is not compulsory. Following code shows use of if-elif-else.
if [conditional 1]:
[Statement 1 to be executed if the conditional 1 evaluates to be True]
elif [conditional 2]:
[Statement 2 to be executed if the conditional 2 evaluates to be True and conditional 1 evaluates to be false]
else:
[Statement 3 to be executed if the conditional evaluates to be conditional 1 and 2 evaluates to be false]
The conditional 1 evaluates to be TRUE then statement 1 gets executed, else if the conditional 2 evaluates to be TRUE then statement 2 gets executed, else statement 3 gets executed (when if and elif are FALSE).
#elif Condition
Num1 = 1000
Num2 = 1000
if Num2 > Num1:
print("Num2 is greater than Num1")
elif Num1 == Num2:
print("Num1 is equal to Num2")
else:
print("Num1 is greater than Num2")
#Output>>>
>>>Num1 is equal to Num2
#If condition is not met
#if condition is False
Num1 = 2000
Num2 = 1000
Num3 = 3000
if Num2 > Num1:
print("Num2 is greater than Num1")
elif Num1 == Num2:
print("Num1 is equal to Num2")
elif Num3 > Num2:
print("Num3 is greater than Num2")
else:
print("Num1 is greater than Num2")
#Output>>>
>>>Num3 is greater than Num2
In the if statement, we are checking the condition. If the condition evaluates as TRUE, then the block of code present inside if will be executed. When the condition becomes FALSE then it will check the elif condition if the condition becomes TRUE, then a block of code present inside the elif statement will be executed. If it is FALSE then a block of code present inside the else statement will be executed.
Nested if Condition
This condition mean that an if statement is present inside another if or if-else block. Python provides this feature as well, this in turn will help us to check multiple conditions in a given program.
if [condition]:
[Statements to execute if condition is true]
if(condition):
#Statements to execute if condition is true
[end of nested if]
[end of if]
The nested if contains another if block inside it.
#nested condition
num = 7
if(num > 0):
print("number is greater then 0")
if(num != 0):
if(num < 10):
print("number is less then 10")
#Output>>>
>>>number is greater then 0
>>>number is less then 10
This will check the first if statement if the condition is TRUE, then the block of code present inside the first if statement will be executed then it will check the second if statement if the first if statement is TRUE and so on.
Wrapping Up
Python conditional statement is quite useful when it comes to decision making in programs to run a certain piece of code based on the values of the conditionals. We have different types of conditional statements like if, if-else, elif, nested if and nested if-else statements which control the execution of our program.
If you have any doubt feel free to leave a comment.
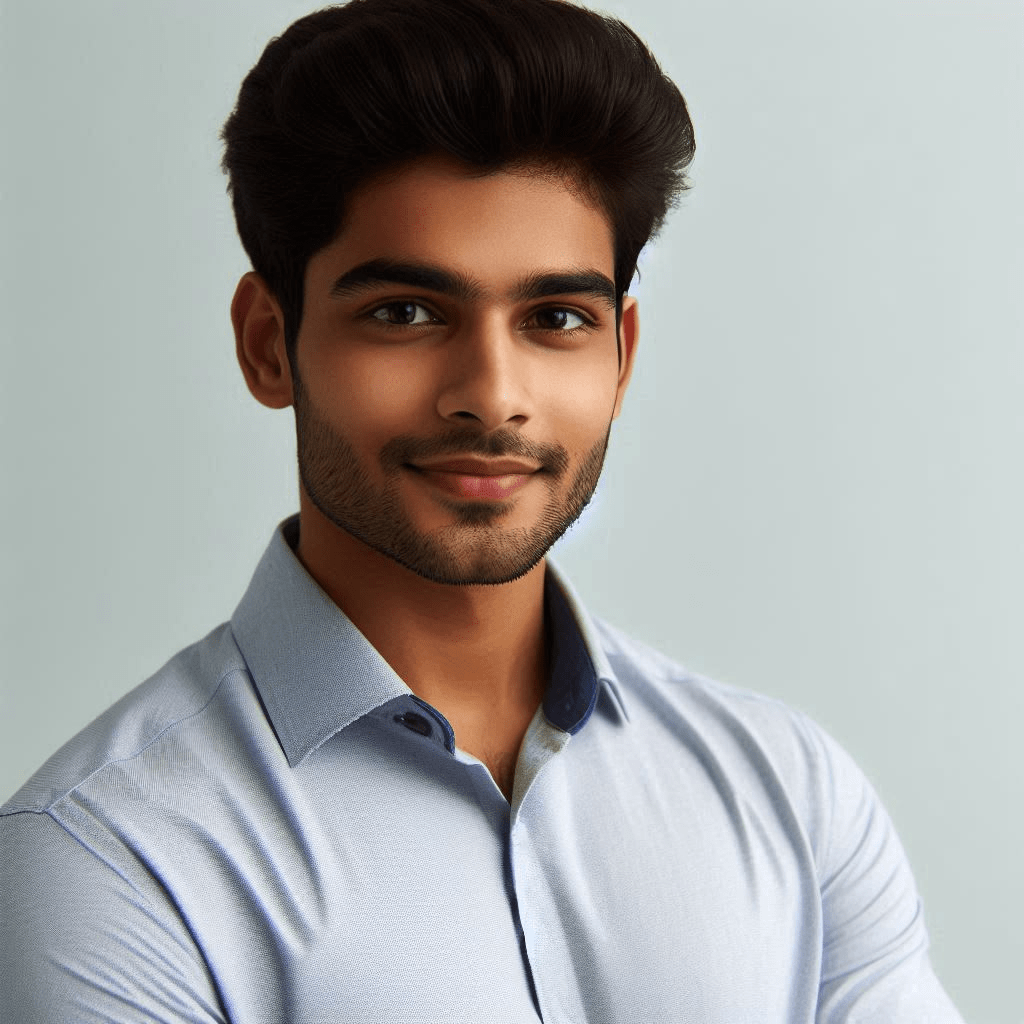
C P Gupta is a YouTuber and Blogger. He is expert in Microsoft Word, Excel and PowerPoint. His YouTube channel @pickupbrain is very popular and has crossed 9.9 Million Views.