The index() method is used to find the position of a particular element in the Python List. Since it is a method of list class, it’s called using a .(dot) operator on list. It checks every element from the starting of the list until it finds a match. The index in python is start from 0.
Syntax
list.index(x[, start[, end]])
Method returns zero-based index element in the list whose value is equal to x. The index()
method only returns the first occurrence of the matching element.
The optional arguments start and end are interpreted as in the slice notation and are used to limit the search to a particular subsequence of the list. In this case, the returned index is computed relative to the beginning of the full sequence rather than the start argument.
Return value from List Index()
- index of first match: It returns the index of the searched element in the list. This method only returns the first occurrence of the matching element.
- ValueError exception: If the searched element is not found in the list, the valueerror exception is raised.
Find the index of the element
If we have a following names of employee list (“Jhon”,”Adwin”,”James”,”Peter”,”Annie”,”Adwin”) and want to search “James” from that list then,
#Employee List
emp_name= ["Jhon","Adwin","James","Peter"]
#index of "James" in Employee List
emp_index = emp_name.index("James")
print("The index of James: ", emp_index)
#Output>>>
The index of James: 2
Index of the element not present in the list
When you try to search the index of an element not present in the list, then it raises a ValueError as follows:
#list of employee name
emp_name= ["Jhon","Adwin","James","Peter","Annie","Adwin"]
#Index of the element is not present in the list
#index of "Kelly" in emp_list
emp_index = emp_name.index("Kelly")
print("The index of Kelly: ", emp_index)
#Output>>
ValueError: 'Kelly' is not in list
Index of Duplicate Values
If the list contains duplicate elements, then the index() function returns index of only first match. In the following example the employee list contains duplicate “Adwin” employee name and if we want to search “Adwin” then it will return 1 i.e. the index of its first occurrence of “Adwin” in the list.
#List contain duplicate elements
emp_name= ["Jhon","Adwin","James","Peter","Annie","Adwin"]
#index will return the position of first element
emp_index = emp_name.index("Adwin")
print("The index of Adwin: ",emp_index)
#Output>>
The index of Adwin: 1
Index of Complex Element
A list can contain other data type like tuple, dictionary and list. Index function can also fetch their position in the list.
complex_list = [(1,2,3,4), {"a" : "b", "c" : "d"}, [True, False]]
index_tuple = complex_list.index((1,2,3,4))
index_dictionary = complex_list.index({"a" : "b", "c" : "d"})
index_list = complex_list.index([True, False])
print("The index of tuple is: ",index_tuple)
print("The index of dictionary is: ",index_dictionary)
print("The index of list is: ",index_list)
Output>>
The index of tuple is: 0
The index of dictionary is: 1
The index of list is: 2
Working of index() With Start and End Parameters
If your list is long and you want to save computational time, then you can provide the start and end index as parameter, where the element will be searched. If you don’t provide the end parameter, it will search till the end parameter.
#Working of index() With Start and End Parameters
#Employee List
emp_name= ["Jhon","Adwin","James","Peter","Annie","Adwin"]
# "Peter" between 1st and 5th index is searched
index = emp_name.index("Peter", 1, 5)
print('The index of Peter: ', index)
#Search "Annie" from 2nd index till end of list
index = emp_name.index("Annie", 2 )
print('The index of Annie: ', index)
#"Jhon" between 2nd and 5th index is searched
index = emp_name.index("Jhon", 2, 5)
print('The index of Jhon: ', index)
#Output>>>
The index of Peter: 3
The index of Annie: 4
Traceback (most recent call last):
File "<string>", line 10, in <module>
ValueError: 'Jhon' is not in list
I hope you this will help you understand index well. If so, leave us comment. And If you have any queries, feel free to comment.
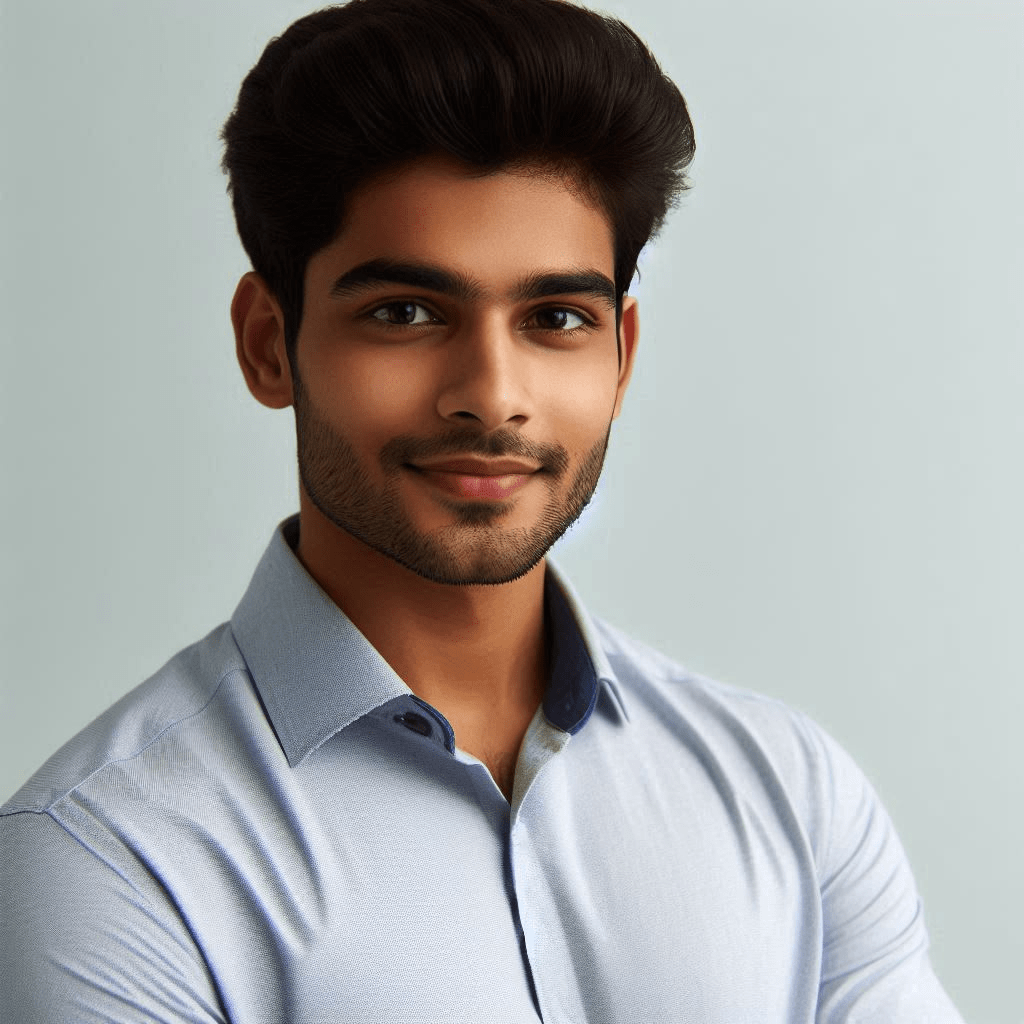
C P Gupta is a YouTuber and Blogger. He is expert in Microsoft Word, Excel and PowerPoint. His YouTube channel @pickupbrain is very popular and has crossed 9.9 Million Views.
Very good blog article. Really looking forward to read more. Really Great!.
Welcome dear.
This is a great tip especially to those new to the blogosphere.
Short but very accurate information… Thanks for sharing this one.
A must read article!